This guide will help you create a WordPress Child Theme, step by step.
Before starting our guide, it’s important to understand what a Child Theme is, and why you need it.
What is a Child Theme?
A child theme inherits the functionality and style of another theme, the parent theme. All themes, excluding child themes, are parent themes.
Parent themes include all of the required template files. For the child theme, the only required file is style.css, but functions.php is recommended to enqueue styles rightly.
Why do I need a WordPress Child Theme?
You have your WordPress site and think it’s time to change it up a bit?
You are creating your dream website with a free theme downloaded from the official WordPress.org repository or a premium theme that you have bought, and do you need to make modifications?
You are a designer or a developer, and do you want to start learning about theme development?
You can use a child theme to change small aspects of your site without losing your customizations when you update the parent theme.
How to create a WordPress Child Theme
Note: all of our free and premium WordPress themes come with a child theme. You can download the zip file in the theme documentation page: https://www.designlabthemes.com/documentation/
First, create a new folder to contain the theme. This goes into the wp-content/themes folder of your WordPress installation. It’s best practice to give a child theme the same name as the parent, but with -child appended to the end.
In the new theme folder, create a stylesheet file named style.css
In this example, we create the child theme of Short News, one of our WordPress themes:
/*
Theme Name: Short News Child Theme
Theme URI: https://www.designlabthemes.com/short-news-wordpress-theme/
Description: Short News Child Theme
Author: Design Lab
Author URI: https://www.designlabthemes.com/
Template: short-news
Version: 1.0.0
License: GNU General Public License v2 or later
License URI: http://www.gnu.org/licenses/gpl-2.0.html
Text Domain: short-news-child
*/
The following information is required:
Theme Name (needs to be unique) and Template (the name of the parent theme directory).
After uploading the new theme folder, go to Appearance » Themes in the WordPress Admin, and look at the Theme Details screen, you would see this:
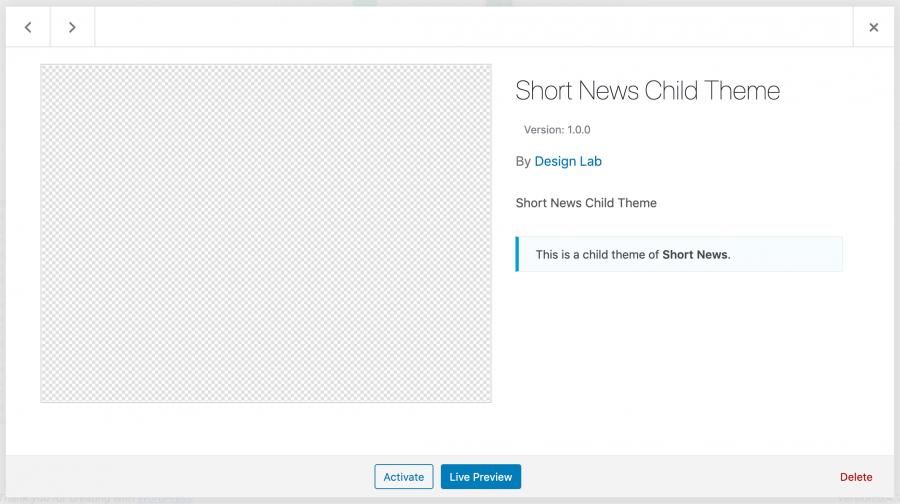
It doesn’t look fine because there is no preview image. Unless the theme is very different from the main theme, just copy the screenshot.png file from the main theme and paste it into the child theme.
Now, create a functions.php file in your child theme’s folder.
The best way of enqueuing the stylesheets is to add a wp_enqueue_scripts action and use wp_enqueue_style() to enqueue a CSS stylesheet.
Note: don’t test the child theme on your website live, please use a staging website or a maintenance mode plugin.
Case 1
If the parent theme loads both stylesheets, the child theme does not need to do anything.
Case 2
If the parent theme loads its style using get_template_directory() or get_template_directory_uri(), the child theme needs to load just the child styles:
/**
* Enqueue the child theme stylesheets.
*/
add_action( 'wp_enqueue_scripts', 'my_theme_enqueue_styles' );
function my_theme_enqueue_styles() {
wp_enqueue_style( 'child-style', get_stylesheet_uri(),
array( 'parenthandle' ), // the parent stylesheet it depends on
wp_get_theme()->get('Version') // this only works if you have Version in the style.css file
);
}
In the code above, we use the function wp_get_theme()->get(‘Version’) to add a version number and prevent browser cache.
Case 3
If the parent theme loads its style using get_stylesheet_directory() or get_stylesheet_uri(), like in our example, the child theme needs to load both parent and child stylesheets.
/**
* Enqueue the parent and child theme stylesheets.
*/
add_action( 'wp_enqueue_scripts', 'short_news_enqueue_styles' );
function short_news_enqueue_styles() {
$theme = wp_get_theme();
wp_enqueue_style( 'short-news-style', get_template_directory_uri() . '/style.css',
array(),
$theme->parent()->get('Version')
);
wp_enqueue_style( 'short-news-child-style', get_stylesheet_uri(),
array( 'short-news-style' ), // the parent stylesheet it depends on
$theme->get('Version')
);
}